Free UI/UX design course
Learn how to create exceptional designs by reading my new tutorial.
Start learningForms
Almost every project needs a form. In this lesson, we will create a contact form and take a closer look at forms as such.
Basic input field
A basic example of the input field consists of the input
element with specified
ID
and label
element connected via this ID
with the
input. Both elements are wrapped in .form-outline
class which provides a material
design look.
Sizing
Set heights using classes like .form-control-lg
and
.form-control-sm
.
Disabled
Add the disabled
boolean attribute on an input to give it a grayed out appearance
and remove pointer events.
Forms
Every group of input fields should reside in a <form>
element. MDB provides
no default styling for the <form>
element, but there are some powerful
browser features that are provided by default.
Since MDB applies display: block
and width: 100%
to almost all our
form controls, forms will by default stack vertically. Additional classes can be used to vary
this layout on a per-form basis.
Login form
A basic example of a simple login form with input fields (email and password), checkbox and submit button.
Checkbox and "forgot password" link are positioned inline by using 2 column grid layout.
Register form
Typical register form with additional register buttons.
Contact form
Typical contact form with textarea input as a message field.
Step 1 - create an empty Contact Section
Let's prepare a place for our form.
In the index.html
file, under the section with pricing cards, add a new section with a grid for 2 columns in the middle.
Step 2 - add contact form
In the first column, add the contact form we presented in this lesson in the example above.
And that's all for this lesson. In the next lesson, we'll finish our Contact section, so when you're ready, click "next".
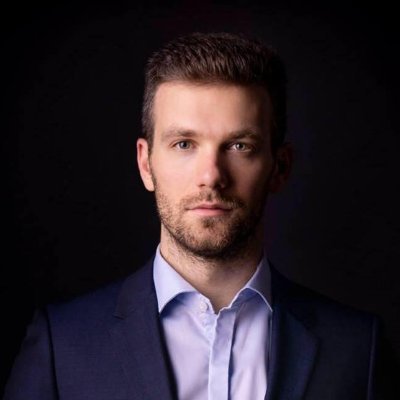
About author
Michal Szymanski
Co Founder at MDBootstrap / Listed in Forbes „30 under 30" / Open-source enthusiast / Dancer, nerd & book lover.
Author of hundreds of articles on programming, business, marketing and productivity. In the past, an educator working with troubled youth in orphanages and correctional facilities.