Chips
Vue Bootstrap 5 Chips component
Responsive chips built with the latest Bootstrap 5 and Vue 3. Chips (aka tags) make it easier to categorize content and browse ie. through different articles from the same category.
MDB tags and chips categorize content with the use of text and icons. Tags and chips make it easier to browse throughout articles, comments or pages. Their main goal is to provide your visitors with an intuitive way of getting what they want. Just consider, how convenient it is to find all the articles related to web development just by using a tag.
Note: Read the API tab to find all available options and advanced customization
Basic example
Chips can be used to represent small blocks of information. They are most commonly used either for contacts or for tags.
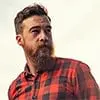
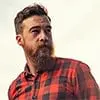
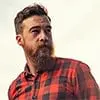
<template>
<MDBChip>Text</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-6.jpg" alt="Contact Person" close>John Doe</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-6.jpg" alt="Contact Person" size="md" close>John Doe
</MDBChip>
<MDBChip src="https://mdbootstrap.com/img/Photos/Avatars/avatar-6.jpg" alt="Contact Person" size="lg" close>John Doe
</MDBChip>
</template>
<script>
import { MDBChip } from 'mdb-vue-ui-kit';
export default {
components: {
MDBChip
}
};
</script>
<script setup lang="ts">
import { MDBChip } from 'mdb-vue-ui-kit';
</script>
Outline
You can use outline styling with outline="color"
attribute.
<template>
<MDBChip outline="primary" close>Primary</MDBChip>
<MDBChip outline="secondary" close>Secondary</MDBChip>
<MDBChip outline="success" close>Success</MDBChip>
<MDBChip outline="danger" close>Danger</MDBChip>
<MDBChip outline="warning" close>Warning</MDBChip>
<MDBChip outline="info" close>Info</MDBChip>
<MDBChip outline="light" close>Light</MDBChip>
<MDBChip outline="dark" close>Dark</MDBChip>
</template>
<script>
import { MDBChip } from 'mdb-vue-ui-kit';
export default {
components: {
MDBChip
}
};
</script>
<script setup lang="ts">
import { MDBChip } from 'mdb-vue-ui-kit';
</script>
Placeholder
Type a name and press enter to add a tag. Click X to remove it.
<template>
<MDBChipsInput label="label"></MDBChipsInput>
</template>
<script>
import { MDBChipsInput } from 'mdb-vue-ui-kit';
export default {
components: {
MDBChipsInput
}
};
</script>
<script setup lang="ts">
import { MDBChipsInput } from 'mdb-vue-ui-kit';
</script>
Initial Value
You can set initial tags with v-model
.
<template>
<MDBChipsInput label="label" v-model="chipsInputValue"></MDBChipsInput>
</template>
<script>
import { MDBChipsInput } from 'mdb-vue-ui-kit';
import { ref } from "vue"
export default {
components: {
MDBChipsInput
},
setup() {
const chipsInputValue = ref(['MDBReact', 'MDBAngular', 'MDBVue', 'MDB5', 'MDB'])
return {
chipsInputValue
}
}
};
</script>
<script setup lang="ts">
import { MDBChipsInput } from 'mdb-vue-ui-kit';
import { ref } from "vue"
const chipsInputValue = ref(['MDBReact', 'MDBAngular', 'MDBVue', 'MDB5', 'MDB'])
</script>
Content Editable
You can set content editable with editable
property.
<template>
<MDBChipsInput label="label" editable></MDBChipsInput>
</template>
<script>
import { MDBChipsInput } from 'mdb-vue-ui-kit';
export default {
components: {
MDBChipsInput
}
};
</script>
<script setup lang="ts">
import { MDBChipsInput } from 'mdb-vue-ui-kit';
</script>
Chips - API
Import
<script>
import {
MDBChip,
MDBChipsInput
} from 'mdb-vue-ui-kit';
</script>
Properties
MDBChip
Name | Type | Default | Description |
---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBChip element. |
Size
|
String | Defines size of the MDBChip element. |
|
outline
|
String | Defines if element should use outline style and which color. | |
close
|
Boolean | false |
Defines if close button should be rendered. |
handleClose
|
Function | Handler for click event on the closing button. |
MDBChipsInput
Name | Type | Default | Description |
---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBChipsInput element. |
id
|
String | uid |
Defines id of the input element inside the MDBChipsInput . |
label
|
String | Defines the label. | |
labelClasses
|
String | Defines classes of the label. | |
placeholder
|
String | Defines the placeholder. | |
secondaryPlaceholder
|
String | Defines the secondary placeholder. | |
chipSize
|
String | Defines the size of the chips elements. | |
editable
|
Boolean | Defines if content of the chips elements is editable. |
Events
MDBChip
Event type | Description |
---|---|
close-chip
|
This event fires when the chip has been closed. |
<template>
<MDBChip outline="primary" @close-chip="doSomething">Primary</MDBChip>
</template>
MDBChipsInput
Event Type | Description |
---|---|
delete-chip
|
This event fires when the chip has been deleted. |
deleted-chip
|
This event fires when the chip has been deleted. Provides the deleted chip as an argument |
add-chip
|
This event fires when the chip has been added. |
added-chip
|
This event fires when the chip has been added. Provides the added chip as an argument |
arrow-down
|
This event fires when the down arrow has been pressed on the chips input. |
arrow-up
|
This event fires when the up arrow has been pressed on the chips input. |
arrow-left
|
This event fires when the left arrow has been pressed on the chips input. |
arrow-right
|
This event fires when the right arrow has been pressed on the chips input. |
<template>
<MDBChipsInput label="label" @delete-chip="doSomething"></MDBChipsInput>
</template>
CSS variables
As part of MDB’s evolving CSS variables approach, chips now uses local CSS variables on
.chip
and .chips
for enhanced real-time customization. Values for
the CSS variables are set via Sass, so Sass customization is still supported, too.
// .chip
--#{$prefix}chip-height: #{$chip-height};
--#{$prefix}chip-line-height: #{$chip-line-height};
--#{$prefix}chip-padding-right: #{$chip-padding-right};
--#{$prefix}chip-margin-y: #{$chip-margin-y};
--#{$prefix}chip-margin-right: #{$chip-margin-right};
--#{$prefix}chip-font-size: #{$chip-font-size};
--#{$prefix}chip-font-weight: #{$chip-font-weight};
--#{$prefix}chip-font-color: #{$chip-font-color};
--#{$prefix}chip-bg: #{$chip-bg};
--#{$prefix}chip-border-radius: #{$chip-br};
--#{$prefix}chip-transition-opacity: #{$chip-transition-opacity};
--#{$prefix}chip-img-margin-right: #{$chip-img-margin-right};
--#{$prefix}chip-img-margin-left: #{$chip-img-margin-left};
--#{$prefix}chip-close-padding-left: #{$chip-close-padding-left};
--#{$prefix}chip-close-font-size: #{$chip-close-font-size};
--#{$prefix}chip-close-opacity: #{$chip-close-opacity};
--#{$prefix}chip-outline-border-width: #{$chip-outline-border-width};
--#{$prefix}chip-md-height: #{$chip-md-height};
--#{$prefix}chip-md-br: #{$chip-md-br};
--#{$prefix}chip-lg-height: #{$chip-lg-height};
--#{$prefix}chip-lg-br: #{$chip-lg-br};
--#{$prefix}chip-contenteditable-border-width: #{$chip-contenteditable-border-width};
--#{$prefix}chip-contenteditable-border-color: #{$chip-contenteditable-border-color};
--#{$prefix}chip-icon-color: #{$chip-icon-color};
--#{$prefix}chip-icon-transition: #{$chip-icon-transition};
--#{$prefix}chip-icon-hover-color: #{$chip-icon-hover-color};
// .chips
--#{$prefix}chips-min-height: #{$chips-min-height};
--#{$prefix}chips-padding-bottom: #{$chips-padding-bottom};
--#{$prefix}chips-margin-bottom: #{$chips-margin-bottom};
--#{$prefix}chips-transition: #{$chips-transition};
--#{$prefix}chips-padding-padding: #{$chips-padding-padding};
--#{$prefix}chips-input-width: #{$chips-input-width};
SCSS variables
$chip-height: 32px;
$chip-md-height: 42px;
$chip-lg-height: 52px;
$chip-font-size: 13px;
$chip-font-weight: 400;
$chip-font-color: $body-color;
$chip-line-height: 2;
$chip-padding-right: 12px;
$chip-br: 16px;
$chip-md-br: 21px;
$chip-lg-br: 26px;
$chip-bg: #eceff1;
$chip-margin-y: 5px;
$chip-margin-right: 1rem;
$chip-transition-opacity: 0.3s linear;
$chip-img-margin-right: 8px;
$chip-img-margin-left: -12px;
$chip-icon-color: #afafaf;
$chip-icon-hover-color: #8b8b8b;
$chip-icon-transition: 0.2s ease-in-out;
$chip-outline-border-width: 1px;
$chip-close-font-size: 16px;
$chip-close-line-height: $chip-height;
$chip-close-padding-left: 8px;
$chip-close-opacity: 0.53;
$chip-contenteditable-border-width: 3px;
$chip-contenteditable-border-color: #b2b3b4;
$chips-margin-bottom: 30px;
$chips-min-height: 45px;
$chips-padding-bottom: 1rem;
$chips-input-font-color: $body-color;
$chips-input-font-size: 13px;
$chips-input-font-weight: 500;
$chips-input-height: $chip-height;
$chips-input-margin-right: 20px;
$chips-input-line-height: $chip-height;
$chips-input-width: 150px;
$chips-transition: 0.3s ease;
$chips-focus-box-shadow: 0.3s ease;
$chips-padding-padding: 5px;