Sticky
Bootstrap 5 Sticky
Sticky is a component which allows elements to be locked in a particular area of the page. It is often used in navigation menus.
Note: Read the API tab to find all available options and advanced customization
*
*
UMD autoinits are enabled
by default. This means that you don't need to initialize
the component manually. However if you are using MDBootstrap ES format then you should pass
the required components to the initMDB
method.
Basic example
To start use sticky just add a .sticky
class and data-mdb-sticky-init
attribute to the element you want to pin.
<a
type="button"
href="https://mdbootstrap.com/docs/standard/getting-started/installation/"
class="btn btn-outline-primary sticky"
data-mdb-sticky-init
data-mdb-ripple-init
>
Download MDB
</a>
// Initialization for ES Users
import { Ripple, Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Sticky });
Sticky bottom
You can pin an element to bottom by adding
data-mdb-sticky-position="bottom"
<img
id="MDB-logo"
src="https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
data-mdb-sticky-init
data-mdb-sticky-position="bottom"
/>
// Initialization for ES Users
import { Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Sticky });
Boundary
Set data-mdb-sticky-boundary="true"
so that sticky only works inside the parent
element. Be sure to set the appropriate height for the parent.
<div style="min-height: 500px" class="text-center">
<img
id="MDB-logo"
src="https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
data-mdb-sticky-init
data-mdb-sticky-boundary="true"
/>
</div>
// Initialization for ES Users
import { Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Sticky });
Outer element as a boundary
You can specify an element selector to be the sticky boundary.
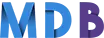
<div style="min-height: 500px" class="d-flex flex-column justify-content-center text-center">
<div>
<img
id="MDB-logo"
src="https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
data-mdb-sticky-init
data-mdb-sticky-boundary="#sticky-stop"
data-mdb-sticky-direction="both"
/>
</div>
<div id="sticky-stop" class="mt-auto" style="height: 5rem">Stop here</div>
</div>
// Initialization for ES Users
import { Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Sticky });
Direction
Default direction of sticky component is down
. You can change it by setting
data-mdb-sticky-direction="up"
or
data-mdb-sticky-direction="both"
<a type="button" class="btn btn-primary sticky" data-mdb-ripple-init data-mdb-sticky-init data-mdb-sticky-boundary="true" data-mdb-sticky-direction="up">Up</a>
<a type="button" class="btn btn-primary sticky" data-mdb-ripple-init data-mdb-sticky-init data-mdb-sticky-boundary="true" data-mdb-sticky-direction="down">Down</a>
<a type="button" class="btn btn-primary sticky" data-mdb-ripple-init data-mdb-sticky-init data-mdb-sticky-boundary="true" data-mdb-sticky-direction="both">Both</a>
// Initialization for ES Users
import { Ripple, Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Sticky });
Animation
You can incorporate an animation that activates when the sticky behavior starts and when the sticky element is
hidden. Specify the CSS class for the animation using the
data-mdb-sticky-animation-sticky
and
data-mdb-sticky-animation-unsticky
attributes.
Remember that not every animation will be appropriate. We suggest using the animations used in the example below.
<img
id="MDB-logo"
src="https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
data-mdb-sticky-init
data-mdb-sticky-animation-sticky="slide-in-down"
data-mdb-sticky-animation-unsticky="slide-up"
data-mdb-sticky-boundary="true"
/>
// Initialization for ES Users
import { Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Sticky });
Sticky - API
Import
Importing components depends on how your application works. If you intend to use the MDBootstrap ES
format, you must
first import the component and then initialize it with the initMDB
method. If you are going to use the UMD
format,
just import the mdb-ui-kit
package.
import { Sticky, initMDB } from "mdb-ui-kit";
initMDB({ Sticky });
import 'mdb-ui-kit';
Usage
Via data attributes
Using the Datatable sticky doesn't require any additional JavaScript code - simply add
data-mdb-Sticky-init
attribute to
.sticky
and use other data attributes to set all options.
For ES
format, you must first import and call the initMDB
method.
<p class="sticky" data-mdb-sticky-init>Example</p>
Via JavaScript
const sticky = document.querySelector('.sticky');
const stickyInstance = new Sticky(sticky);
const sticky = document.querySelector('.sticky');
const stickyInstance = new mdb.Sticky(sticky);
Via jQuery
Note: By default, MDB does not include jQuery and you have to add it to the project on your own.
$(document).ready(() => {
$('.sticky').sticky();
});
Options
Options can be passed via data attributes or JavaScript. For data attributes, append the option name to
data-mdb-
, as in data-mdb-sticky-delay="50"
.
Name | Type | Default | Description |
---|---|---|---|
stickyActiveClass
|
String | '' |
Set custom active class |
stickyAnimationSticky
|
String | '' |
Set sticky animation |
stickyAnimationUnsticky
|
String | '' |
Set unsticky animation |
stickyBoundary
|
Boolean | String | false |
set to true to stop sticky on the end of the parent |
stickyDelay
|
Number | 0 |
Set the number of pixels beyond which the item will be pinned |
stickyDirection
|
String | 'down' |
Set the scrolling direction for which the element is to be sticky. Available values: up , down , both |
stickyMedia
|
Number | 0 |
Set the minimum width in pixels for which the sticky should work |
stickyOffset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
stickyPosition
|
String | 'top' |
Set the edge of the screen the item should stick to |
Methods
Name | Description | Example |
---|---|---|
dispose |
Removes mdb.Sticky instance. |
sticky.dispose()
|
activate |
Start sticky manually. |
sticky.activate()
|
deactivate
|
Stop sticky manually. |
sticky.deactivate()
|
getInstance
|
Static method which allows you to get the sticky instance associated to a DOM element. |
Sticky.getInstance(myStickyEl)
|
getOrCreateInstance
|
Static method which returns the sticky instance associated to a DOM element or create a new one in case it wasn't initialized. |
Sticky.getOrCreateInstance(myStickyEl)
|
const myStickyEl = document.getElementById('sticky');
const sticky = new Sticky(myStickyEl);
sticky.deactivate();
const myStickyEl = document.getElementById('sticky')
const sticky = new mdb.Sticky(myStickyEl)
sticky.deactivate();
Events
Name | Description |
---|---|
activated.mdb.sticky
|
This event is emitted after elements is sticked to the edge of a specific area. |
deactivated.mdb.sticky
|
This event is emitted when elements detach from the edge of a specific area. |
const myStickyEl = document.getElementById('sticky')
myStickyEl.addEventListener('activated.mdb.sticky', (e) => {
// do something...
})