Onboarding
Angular Bootstrap 5 Onboarding plugin
Onboarding is a guide plugin to show users how to use your website
Angular Onboarding plugin built with the latest Bootstrap 5. It is is a guide plugin to show users how to use your website.Note: Read the API tab to find all available options and advanced customization
Basic example
To initialize Onboarding on your page simply add anchors with mdbOnboardingAnchor
directive to the
elements of your steps.
As a first parameter to the init()
method specify steps by giving proper
id
corresponding to previously added anchors, content
of a step, and other individual options.
As a second parameter you can pass global options which will be introduced to every step. Note that individual options have higher priority than global options.
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button
class="btn btn-danger"
(click)="handleClick()"
>
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p
[mdbOnboardingAnchor]="'third-step'"
class="card-text"
>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init([
{ id: 'first-step', content: 'This button has just started your onboarding' },
{
id: 'second-step',
content: 'This is just basic example of initial onboarding options and configurations',
},
{ id: 'third-step', content: 'There is many more options in the examples below' },
]);
this.onboardingService.start();
}
}
Start options
Autostart
Onboarding can be auto initialized by passing
delay
option with the value of seconds after which Onboarding will be triggered
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<button
class="btn btn-danger"
(click)="handleClick()"
>
Start onboarding
</button>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div #autostartTrigger class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-autostart'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-autostart'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p
[mdbOnboardingAnchor]="'third-step-autostart'"
class="card-text"
>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{ id: 'first-step-autostart', content: 'Example of autostarted onboarding experience' },
{
id: 'second-step-autostart',
content: 'Pass delay time in seconds to autostart onboarding',
},
{
id: 'third-step-autostart',
content: 'Delay time will also work with other starting options',
},
], { delay: 3 }
);
this.onboardingService.start();
}
}
Event triggered start
Run initialization methods on any trigger.
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button class="btn btn-danger" (mouseover)="handleMouseover()">
Hover over me to start
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-hover-trigger'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-hover-trigger'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p
class="card-text"
>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleMouseover() {
this.onboardingService.init([
{
id: 'first-step-hover-trigger',
content: "You can use events such as 'click' or 'mouseover'",
},
{
id: 'second-step-hover-trigger',
content: "You can even use 'load' event on a window element!",
},
]);
this.onboardingService.start();
}
}
Backdrop options
Global backdrop
Backdrop for every step inside Onboarding can be set via
backdrop
option globally, same as the backdropOpacity
option which sets an opacity.
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<button class="btn btn-danger" (click)="handleClick()">Start onboarding</button>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-backdrop-global'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-backdrop-global'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a
[mdbOnboardingAnchor]="'third-step-backdrop-global'"
href="#!"
role="button"
>
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-backdrop-global',
content: 'Backdrop is set to false by default',
},
{
id: 'second-step-backdrop-global',
content:
'Backdrop options set in Onboarding container will be passed to each step element inside it',
},
{
id: 'third-step-backdrop-global',
content:
'If you want to set global backdrop but turn it off for one step use options for individual step from the next example',
},
],
{
backdrop: true,
backdropOpacity: 0.3,
}
);
this.onboardingService.start();
}
}
Individual step backdrop
Indivudal options have higher priority than global options.
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<button class="btn btn-danger" (click)="handleClick()">Start onboarding</button>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-backdrop-individual'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-backdrop-individual'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a
[mdbOnboardingAnchor]="'third-step-backdrop-individual'"
href="#!"
role="button"
>
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-backdrop-individual',
content:
'Individual step options have higher specificity than global. Setting backdrop to a single step will override its global backdrop options',
backdrop: true,
backdropOpacity: 0.6,
},
{
id: 'second-step-backdrop-individual',
content: 'This element has backdrop with opacity 0.2',
backdrop: true,
backdropOpacity: 0.2,
},
{
id: 'third-step-backdrop-individual',
content: 'And this element has backdrop with opacity 0.8',
backdrop: true,
backdropOpacity: 0.8,
},
],
{
backdropOpacity: 0.8,
}
);
this.onboardingService.start();
}
}
Autoplay
Set autoplay
and stepDuration
to enable autoplay
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<button class="btn btn-danger" (click)="handleClick()">Start onboarding</button>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-autoplay'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-autoplay'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p [mdbOnboardingAnchor]="'third-step-autoplay'" class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a
[mdbOnboardingAnchor]="'fourth-step-autoplay'"
href="#!"
role="button"
>
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-autoplay',
content: 'This step has globally set duration for 2 seconds',
},
{
id: 'second-step-autoplay',
content:
'This step has overriden duration with data-mdb-duration attribute set to 4 seconds',
stepDuration: 4,
},
{
id: 'third-step-autoplay',
content:
'This step has data-mdb-autoplay indivudally set to false. Click next or use arrow to open next step',
autoplay: false,
},
{
id: 'fourth-step-autoplay',
content: 'This step will automatically close after 5 seconds',
stepDuration: 5,
},
],
{ autoplay: true, stepDuration: 2 }
);
this.onboardingService.start();
}
}
Autoscroll
Toggle auto scrolling into element that is outside the view with
autoscroll
option.
<section
class="border p-4 d-flex justify-content-center onboarding"
>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button class="btn btn-danger" (click)="handleClick()">
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<div class="row d-flex justify-content-center">
<div
style="margin-bottom: 150vh;"
[mdbOnboardingAnchor]="'first-step-autoscroll'"
>
Scroll from this
</div>
<div
[mdbOnboardingAnchor]="'second-step-autoscroll'"
>
... to that
</div>
</div>
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-autoscroll',
content: 'Autoscroll is set to true by default',
},
{
id: 'second-step-autoscroll',
content:
'To disable it for single step use autoscroll: false; option on that step',
},
],
{ backdrop: true }
);
this.onboardingService.start();
}
}
Popover customization
Popover class
Add custom class for popovers by setting customClass
option and style it in
your css sheet.
<section
class="border p-4 d-flex justify-content-center onboarding"
>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button class="btn btn-danger" (click)="handleClick()">
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'first-step-custom-class'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4
[mdbOnboardingAnchor]="'second-step-custom-class'"
class="card-title"
>
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a [mdbOnboardingAnchor]="'third-step-custom-class'"
href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a
href="#!"
role="button"
>
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-custom-class',
content: 'Globally changed button labels',
customClass: 'custom-class-individual',
},
{
id: 'second-step-custom-class',
content: 'Locally changed control labels',
},
{
id: 'third-step-custom-class',
content: 'You can even use our icons inside button labels!',
},
],
{ customClass: 'custom-class-global' }
);
this.onboardingService.start();
}
}
Container example
Gallery
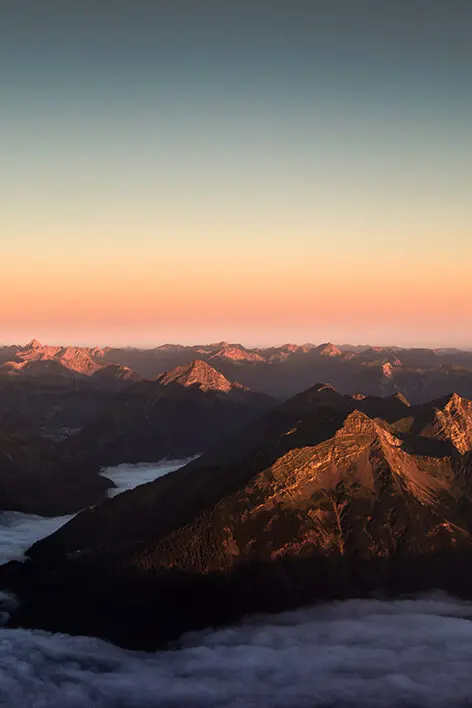
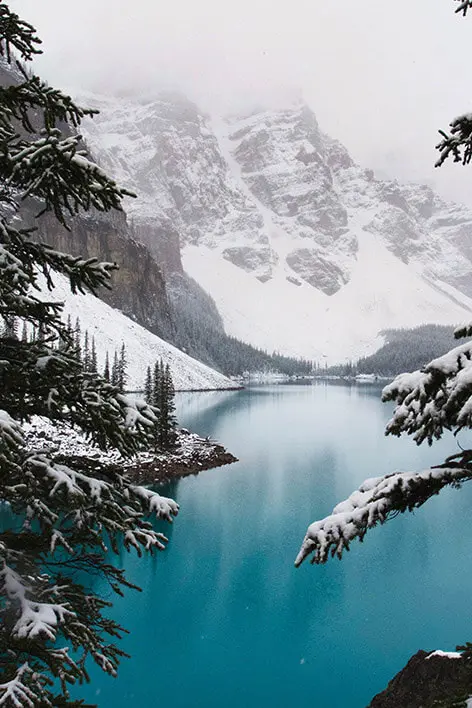
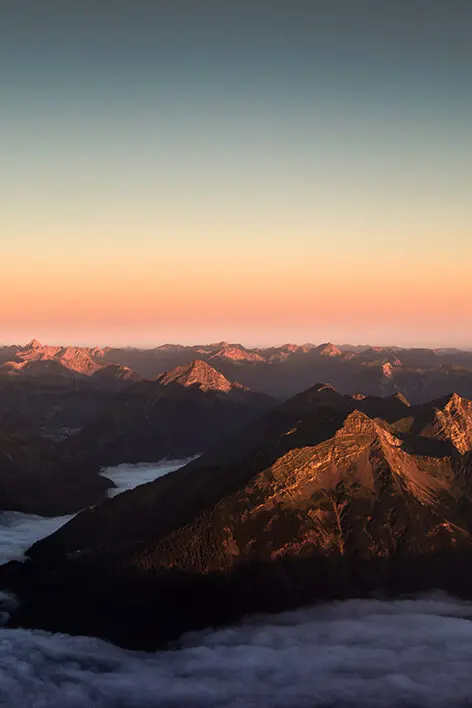
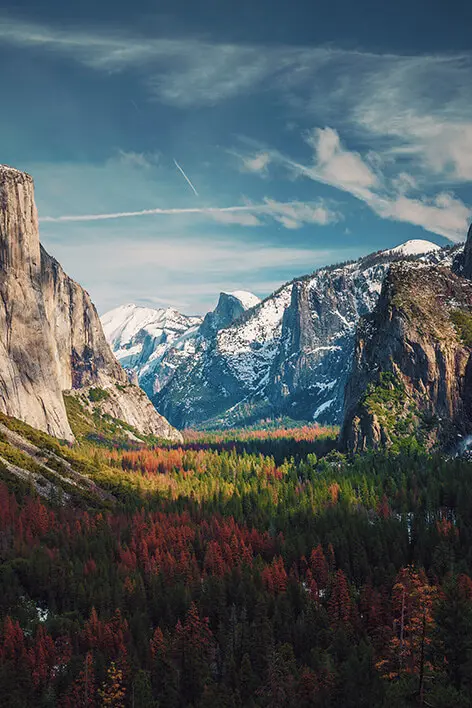
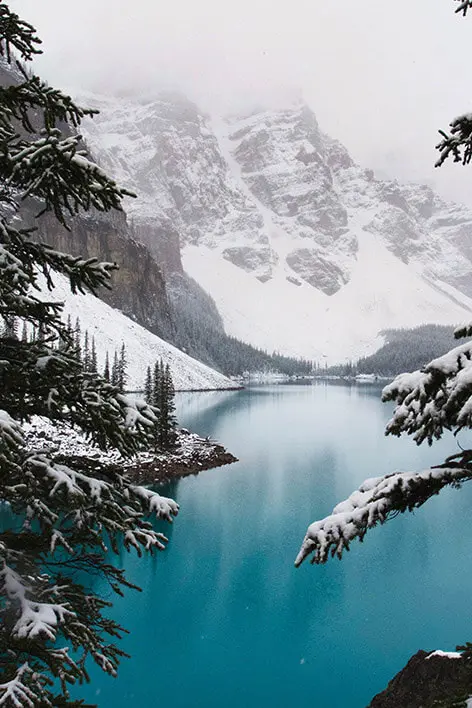
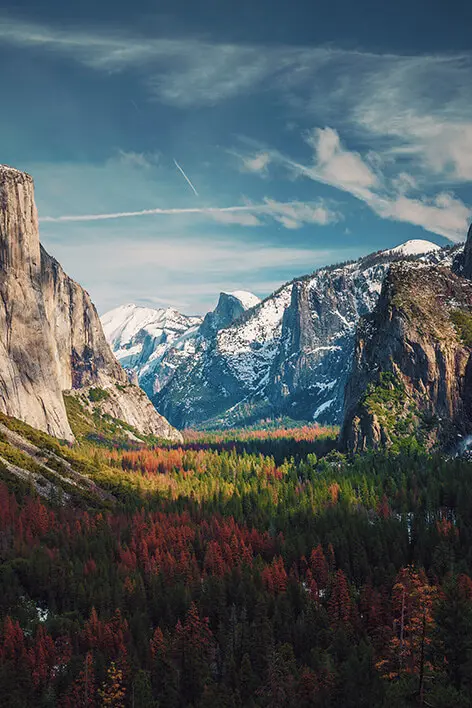
<section
class="border p-4 d-flex justify-content-center onboarding"
style="max-height: 120vh; overflow-y: auto;"
#onboardingContainer
>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button
(click)="handleClick()"
class="btn btn-danger"
[mdbOnboardingAnchor]="'first-step-container'"
id="container-starter"
>
Watch Demo <i class="fas fa-eye"></i>
</button>
</div>
</div>
<hr />
<!-- Cards container -->
<div class="row my-4">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>John Doe</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>Kate Smith</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Graphic designer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>Natalie Code</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Backend developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
<!-- Cards container -->
<hr />
<h4 class="text-center my-4"
[mdbOnboardingAnchor]="'third-step-container'"
>
Gallery
</h4>
<!-- Gallery -->
<div
class="row"
[mdbOnboardingAnchor]="'fourth-step-container'"
>
<!-- Grid row -->
<div class="my-3 row">
<!-- Grid column -->
<div class="mb-3 col-md-4">
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain2.webp"
alt="Mountains in the Clouds"
/>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="mb-3 col-md-4">
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain1.webp"
alt="Wintry Mountain Landscape"
/>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="mb-3 col-md-4">
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain2.webp"
alt="Mountains in the Clouds"
/>
</div>
<!-- Grid column -->
</div>
<!-- Grid row -->
<!-- Grid row -->
<div class="my-3 row">
<!-- Grid column -->
<div
class="mb-3 col-md-4"
[mdbOnboardingAnchor]="'second-step-container'"
>
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain3.webp"
alt="Yosemite National Park"
/>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="mb-3 col-md-4">
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain1.webp"
alt="Wintry Mountain Landscape"
/>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="mb-3 col-md-4">
<img
class="img-fluid"
src="https://mdbcdn.b-cdn.net/img/Photos/Vertical/mountain3.webp"
alt="Yosemite National Park"
/>
</div>
<!-- Grid column -->
</div>
<!-- Grid row -->
</div>
<!-- Gallery -->
<!-- Cards container -->
<div class="row my-4"
[mdbOnboardingAnchor]="'fifth-step-container'"
>
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>John Doe</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>Kate Smith</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Graphic designer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title"><strong>Natalie Code</strong></h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Backend developer</h6>
<!-- Text -->
<p class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
<!-- Cards container -->
</div>
</section>
import { Component, ElementRef, ViewChild } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
@ViewChild('onboardingContainer') onboardingContainer!: ElementRef<any>;
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init(
[
{
id: 'first-step-container',
content: 'Backdrop is set to false by default',
},
{
id: 'second-step-container',
content:
'Backdrop options set in Onboarding container will be passed to each step element inside it',
},
{
id: 'third-step-container',
content: 'Backdrop is set to false by default',
},
{
id: 'fourth-step-container',
content: 'Backdrop is set to false by default',
},
{
id: 'fifth-step-container',
content: 'Backdrop is set to false by default',
},
],
{ backdrop: true, container: this.onboardingContainer }
);
this.onboardingService.start();
}
}
Index example
<section class="border p-4 d-flex justify-content-center">
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button
class="btn btn-danger"
(click)="handleClick()"
[mdbOnboardingAnchor]="'first-step-index'"
>
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'second-step-index'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title">
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p
[mdbOnboardingAnchor]="'third-step-index'"
class="card-text"
>
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex,
recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button"
[mdbOnboardingAnchor]="'fourth-step-index'"
>
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
</section>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init([
{ id: 'first-step-index', content: 'This is the first step in the object' },
{
id: 'second-step-index',
content: 'This is the second step in the object',
index: 3,
},
{ id: 'third-step-index', content: 'This is the third step in the object', index: 2 },
{ id: 'fourth-step-index', content: 'This is the fourth step in the object', index: 2 },
]);
this.onboardingService.start();
}
}
Onboarding - API
Installation
To install and configure the plugin follow our Plugins Installation Guide. Please remember to update all the plugin names and import paths. You can find all the necessary information in the Import section.
npm i git+https://oauth2:ACCESS_TOKEN@git.mdbootstrap.com/mdb/angular/mdb5/plugins/prd/onboarding
Import
import { MdbOnboardingModule } from 'mdb-angular-onboarding';
…
@NgModule ({
...
imports: [MdbOnboardingModule],
...
})
Inputs
MdbOnboardingDirective
Name | Type | Default | Description |
---|---|---|---|
mdbOnboardingAnchor
|
string | '' |
Marks the element as an anchor to be referenced later in an id option |
Outputs
MdbOnboardingService
Name | Type | Description |
---|---|---|
onboardingStart
|
EventEmitter<void> | Emitted when an Onboarding has started. |
onboardingEnd
|
EventEmitter<void> | Emitted when the last step of the Onboarding has ended. |
onboardingOpen
|
EventEmitter<void> | Emitted when a step element has opened. |
onboardingClose
|
EventEmitter<void> | Emitted when a step element has closed |
onboardingNext
|
EventEmitter<void> | Emitted when next step is about to be opened. |
onboardingPrev
|
EventEmitter<void> | Emitted when previous step is about to be opened. |
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button
class="btn btn-danger"
(click)="handleClick()"
[mdbOnboardingAnchor]="'first-step'"
>
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'second-step'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title">
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p [mdbOnboardingAnchor]="'third-step'" class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
import { Component, OnInit } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent implements OnInit {
constructor(public onboardingService: MdbOnboardingService) {}
ngOnInit(): void {
this.onboardingService.onboardingStart.subscribe(() =>
console.log('on onboarding start')
);
}
handleClick() {
this.onboardingService.init([
{
id: 'first-step',
content: 'This button has just started your onboarding',
},
{
id: 'second-step',
content:
'This is just basic example of initial onboarding options and configurations',
},
{
id: 'third-step',
content: 'There is many more options in the examples below',
},
]);
this.onboardingService.start();
}
}
Methods
MdbOnboardingService
Name | Description | Example |
---|---|---|
init(steps, config?) |
Initializes the Onboarding with given steps: MdbOnboardingStep[] and config: MdbOnboardingConfig .
|
onboardingService.init(steps, options);
|
close |
Closes opened step popover. |
onboardingService.close()
|
nextStep
|
Opens next step popover. |
onboardingService.nextStep()
|
prevStep
|
Opens previous step popover. |
onboardingService.prevStep()
|
pause
|
Pauses Onboarding on current step when autoplay is on. |
onboardingService.pause()
|
resume |
Resumes Onboarding on current step when autoplay is on. |
onboardingService.resume()
|
<div class="container">
<div class="row justify-content-center">
<div class="col-md-3 text-center">
<button
class="btn btn-danger"
(click)="handleClick()"
[mdbOnboardingAnchor]="'first-step'"
>
Start onboarding
</button>
</div>
</div>
<hr />
<div class="row d-flex justify-content-center">
<!-- Card Regular -->
<div class="col-md-4">
<div class="card">
<!-- Card image -->
<img
[mdbOnboardingAnchor]="'second-step'"
class="card-img-top"
src="https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp"
alt="Man in Cap and Glasses"
/>
<a>
<div class="mask rgba-white-slight"></div>
</a>
<!-- Card content -->
<div class="card-body text-center">
<!-- Title -->
<h4 class="card-title">
<strong>John Doe</strong>
</h4>
<!-- Subtitle -->
<h6 class="fw-bold indigo-text py-2">Web developer</h6>
<!-- Text -->
<p [mdbOnboardingAnchor]="'third-step'" class="card-text">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam.
</p>
<div class="d-flex justify-content-evenly">
<!-- Facebook -->
<a href="#!" role="button">
<i class="fab fa-facebook-f fa-lg"></i>
</a>
<!-- Twitter -->
<a href="#!" role="button">
<i class="fab fa-twitter fa-lg"></i>
</a>
<!-- Google + -->
<a href="#!" role="button">
<i class="fab fa-dribbble fa-lg"></i>
</a>
</div>
</div>
</div>
</div>
<!-- Card Regular -->
</div>
</div>
import { Component } from '@angular/core';
import { MdbOnboardingService } from 'mdb-angular-onboarding';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
constructor(public onboardingService: MdbOnboardingService) {}
handleClick() {
this.onboardingService.init([
{
id: 'first-step',
content: 'This button has just started your onboarding',
},
{
id: 'second-step',
content:
'This is just basic example of initial onboarding options and configurations',
},
{
id: 'third-step',
content: 'There is many more options in the examples below',
},
]);
this.onboardingService.start();
}
}
Advanced types
MdbOnboardingConfig
Name | Type | Default | Description |
---|---|---|---|
backdrop
|
boolean | undefined | false |
Defines whether backdrop should be rendered |
backdropOpacity
|
number | undefined | 0.4 |
Defines the backdrop opacity |
nextLabel
|
string | undefined | 'Next' |
Overwrites label of the 'Next' button |
prevLabel
|
string | undefined | 'Back' |
Overwrites label of the 'Back' button |
skipLabel
|
string | undefined | 'Skip' |
Overwrites label of the 'Skip' button |
finishLabel
|
string | undefined | 'Finish' |
Overwrites label of the 'Finish' button |
pauseLabel
|
string | undefined | 'Pause' |
Overwrites label of the 'Pause' button |
resumeLabel
|
string | undefined | 'Resume' |
Overwrites label of the 'Resume' button |
btnNextClass
|
string | undefined | 'btn-primary' |
Overwrites class of the 'Next' button |
btnPrevClass
|
string | undefined | 'btn-primary' |
Overwrites class of the 'Back' button |
btnSkipClass
|
string | undefined | 'btn-danger' |
Overwrites class of the 'Skip' button |
btnFinishClass
|
string | undefined | 'btn-danger' |
Overwrites class of the 'Finish' button |
btnPauseClass
|
string | undefined | 'btn-primary' |
Overwrites class of the 'Pause' button |
btnResumeClass
|
string | undefined | 'btn-success' |
Overwrites class of the 'Resume' button |
customClass
|
string | undefined | '' |
Custom class for the popover |
autoplay
|
boolean | undefined | false |
Defines whether autoplay is on or off |
stepDuration
|
number | undefined | 4 |
Defines the duration of a step for the autoplay in seconds |
autoscroll
|
boolean | undefined | true |
Defines whether window should automatically scroll into opened step position |
placement
|
'top' | 'right' | 'bottom' | 'left' | undefined | 'bottom' |
Defines placement of step popover |
container
|
ElementRef | null | undefined | null |
Defines wrapper element for onboarding |
delay
|
number | undefined | undefined |
Defines time in seconds after which Onboarding will start. |
index
|
number | undefined | 1 |
Defines index number for step element |
MdbOnboardingStep
Note: Due to its complexity, we present this advanced type in the form of a snippet rather than a table.
export interface MdbOnboardingStep {
id: string;
content: string;
backdrop?: boolean;
backdropOpacity?: number;
nextLabel?: string;
prevLabel?: string;
skipLabel?: string;
finishLabel?: string;
pauseLabel?: string;
resumeLabel?: string;
btnNextClass?: string;
btnPrevClass?: string;
btnSkipClass?: string;
btnFinishClass?: string;
btnPauseClass?: string;
btnResumeClass?: string;
customClass?: string | string[];
autoplay?: boolean;
stepDuration?: number;
autoscroll?: boolean;
placement?: 'top' | 'right' | 'bottom' | 'left';
container?: ElementRef<any> | null;
delay?: number;
index?: number;
}